Initial Answers
This example demonstrates how to pre-populate the Wizard.
Code
vue
<script setup lang='ts'>
import { z } from 'zod'
import Wizard, { type Form } from 'wizard-of-zod'
const forms: Form<z.ZodObject<any>>[] = [
{
schema: z.object({
givenName: z.string().min(2),
familyName: z.string()
})
},
{
schema: z.object({
gender: z.enum(['male', 'female'])
})
},
{
schema: z.object({
age: z.number()
})
},
]
const handleCompleted = (data: Record<string, any>) => {
console.log(data)
}
</script>
<template>
<div class="h-screen flex justify-center items-center">
<Wizard
:classes="{
woz: 'w-1/3',
wozForm: 'space-y-8',
}"
:forms="forms"
:initial-answers="{
givenName: 'John',
familyName: 'Doe'
}"
@completed="handleCompleted"
/>
</div>
</template>
INFO
The wrapping <div>
and classes
prop are just used here for presentation purposes. You should omit them or use your own values.
Screenshot
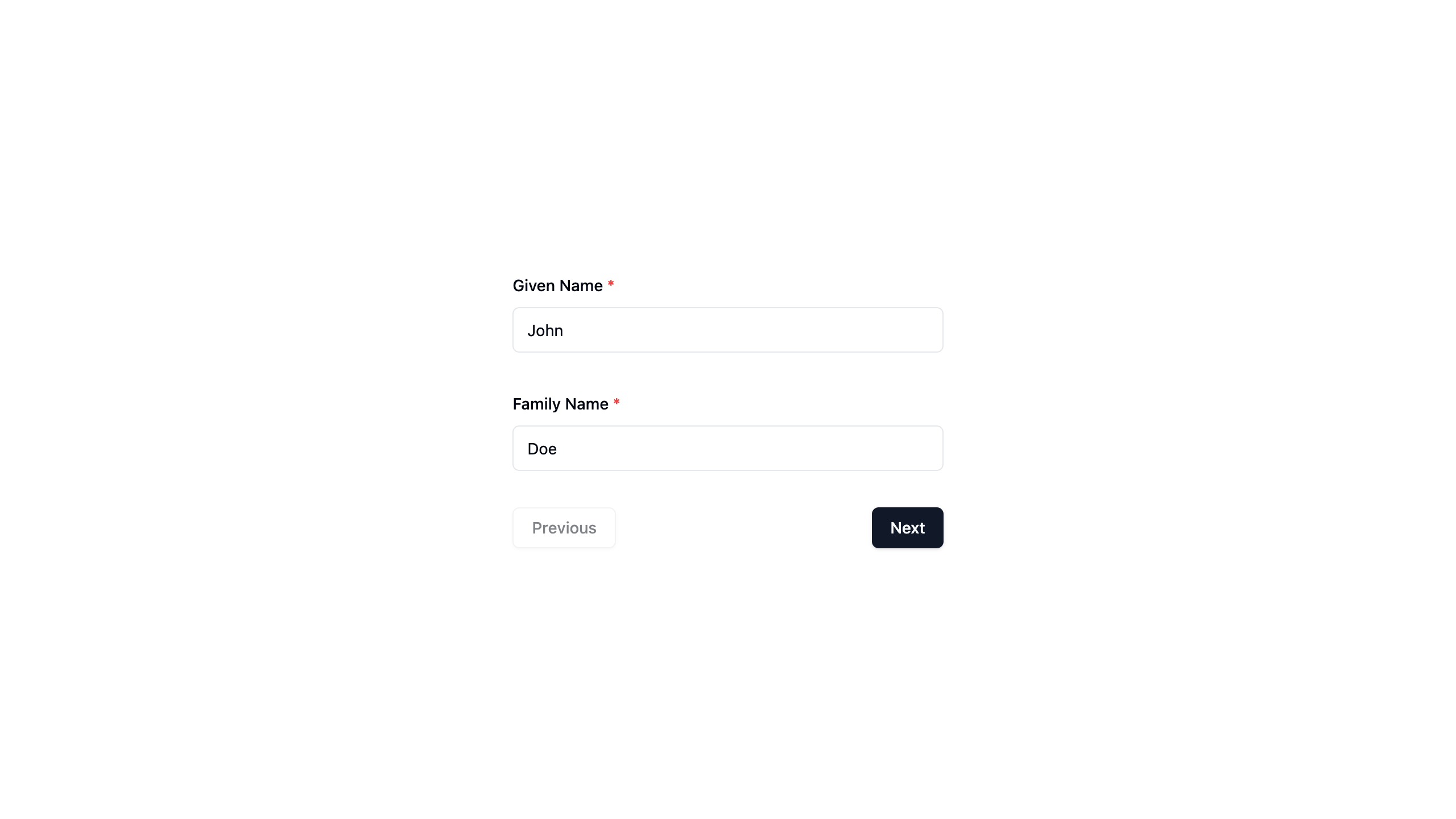
Resulting Data
javascript
{
givenName: 'John',
familyName: 'Doe',
gender: 'male',
age: 44
}