Kitchen Sink
The following "Kitchen Sink" example demonstrates almost a bit of everything in terms of props customisation.
Code
vue
<script setup lang='ts'>
import { z } from 'zod'
import Wizard, { type Form } from 'wizard-of-zod'
const forms: Form<z.ZodObject<any>>[] = [
{
schema: z.object({
givenName: z.string().describe('What is your given name?'),
familyName: z.string().describe('What is your family name?'),
gender: z.enum(['male', 'female']).describe('What is your gender?')
}),
fieldConfig: {
givenName: {
inputProps: {
readonly: true
}
}
}
},
{
title: 'Contact Details',
description: 'We ask for these so we can contact you if we need to.',
schema: z.object({
email: z.string().describe('What is your email address?'),
phone: z.string().describe('What is your phone number?')
})
}
]
const initialAnswers = {
givenName: 'John',
email: 'john.doe@example.com'
}
const handleCompleted = (data: Record<string, any>) => {
console.log(data)
}
</script>
<template>
<div class="h-screen flex justify-center items-center">
<Wizard
:classes="{
woz: 'w-1/3',
wozForm: 'space-y-8',
wozPreview: 'gap-4'
}"
:custom-i18n="{
next: 'Continue',
previous: 'Go Back'
}"
:forms="forms"
:initial-answers="initialAnswers"
preview="table"
progress-indicator="stepper"
@completed="handleCompleted"
/>
</div>
</template>
INFO
The wrapping <div>
and classes
prop are just used here for presentation purposes. You should omit them or use your own values.
Screenshot
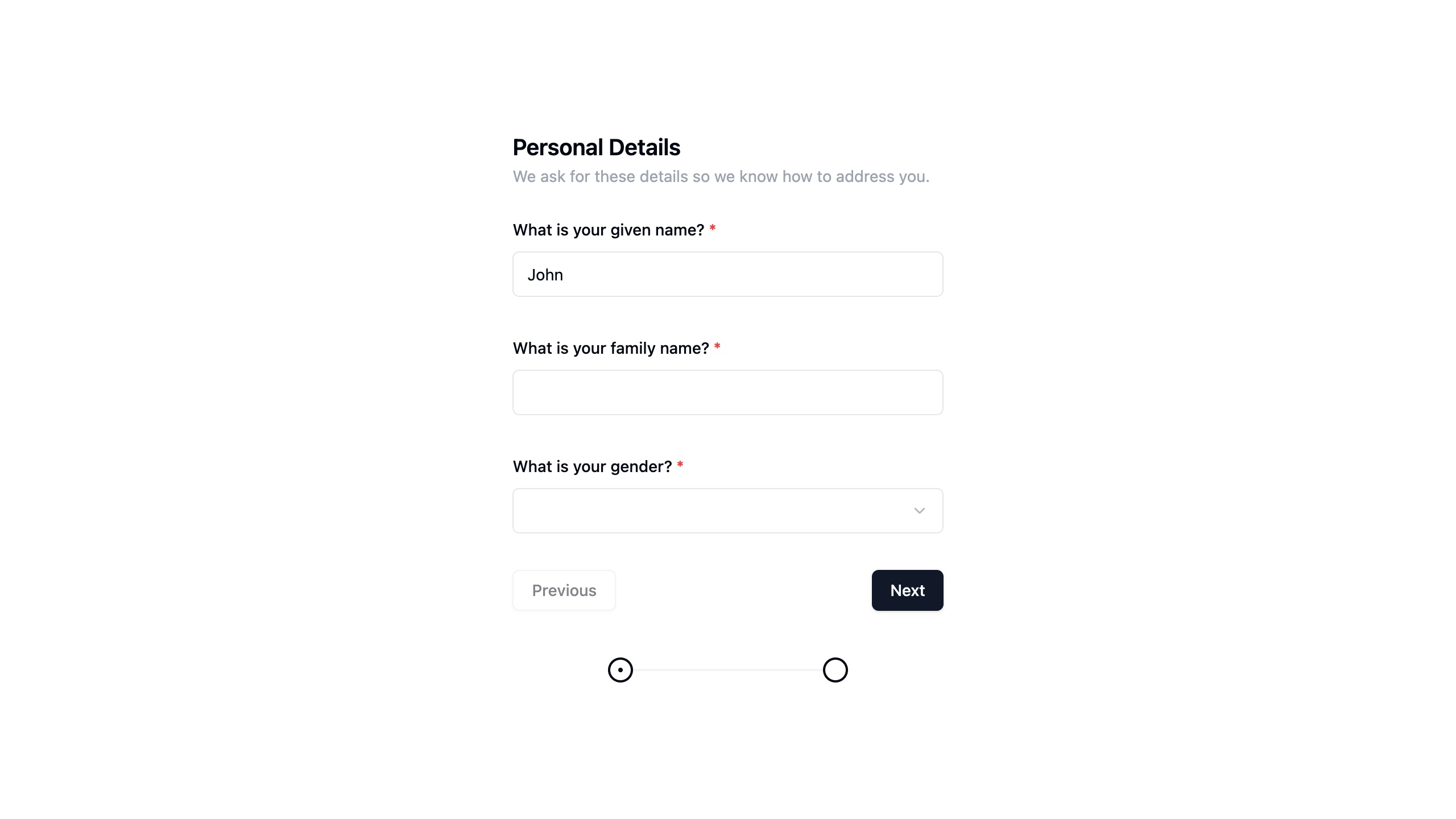
Resulting Data
javascript
{
givenName: 'John',
familyName: 'Doe',
gender: 'male',
email: 'john.doe@example.com',
phone: '+1234567890'
}